Examples 41-50¶
Example 41 - Scorpion Watchdog keep system running¶
This example documents how to communicate with the Resuscitator program to create a watchdog for both Scorpion and a Proscilica camera which is controlled by Scorpion.
- The Resuscitator program - also named Scorpion Watchdog - is a program managing tool and is available on the Scorpion CD. * This program can control startup, shutdowns sequence and be a watchdog for all kinds of programs.
- This example shows how to use it to implement a fast watchdog if a program stops capturing images.
Requirements:
- Python
- Python for Windows Extentions
- Resuscitator
Scorpion is started and controlled by Resuscitator and must be closed when it is started from Resuscitator.
- The Resuscitator configuration file - Resuscitator.ini - is placed in the profile base directory.
Scorpion and Resuscitator will communicate as follows:
Scorpion will write two lines in Resuscitator.ini file. They are stated below
[AliveStatus]
status= 1
In our example Resuscitator will check if these two lines has been written every 10 seconds.
- If they are present in the file, Resuscitator will delete them.
- If not, Resuscitator will restart scorpion. It is important that Scorpion writes the program lines above more frequently than they are written (for instance every second)
Scorpion Setup:
Central Start
def KickResuscitatior(applicationName, iniFileName)
import win32api
profile = GetStringValue('System.Profile')
# when the code is executed, profile will contain a string with the the path of the current scorpion profile.
win32api.WriteProfileVal('AliveStatus',applicationName,1,profile + '\\' + iniFileName)
#for writing the lines to the file using the win32api (for details read the win32 api)
The script which calls the KickResuscitator function will must be executed both when the Scorpion is IDLE, and when Scorpion is RUNNING. If RUNNING we call Grab action, which gets a snapshot from the camera. When the camera has sent the snapshot, the action AfterInspect will be trigged. In AfterInspect we’ll call the KickResuscitator function, which then only is trigged if the camera is working properly. In sytem state idle, we won’t check if the camera is operating correctly, and call the KickResuscitator function directly. We will use Scorpion actions and events to enable this. For more information on Scorpion actions and events, klick here.
This example implements the strategy above by defining two actions in the actions tab, and one event in the scheduler: CameraTrigger and AfterInspect. After inspect is a system defined event, and should be filled with one new command; command = Script, parameters= KickResuscitator(‘Scorpion’,’ resuscitator.ini’). In the camera trigger action we have added two new commands. The first one has the command=Grab and a guard=running. The second one has command = Script, parameters= KickResuscitator(‘Scorpion’,’ resuscitator.ini’), and guard = Inverted running. In the scheduler create a new Event. Give it the following attributes; name: nextImage, command: cameraTrigger, frequency: ‘every nth millisecond’, period: 200
Resuscitator Setup:
Place a shortcut to Resuscitator in the startup group, edit the properties:
Target : C:\Program Files\Tordivel AS\Resuscitator for NT\FindResuscitator.exe
Start in: "C:\Program Files\Tordivel AS\Scorpion 7\Test Prosilica Watchdog
In the Resuscitator program press configure button, and edit the properties for each application:
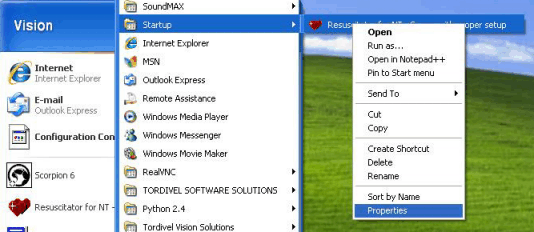
Under configure for applications: Shutdown
Program path: C:\Program Files\TORDIVEL AS\Resuscitator for NT\SHUTDOWN.EXE
Start in: C:\Program Files\TORDIVEL AS\Resuscitator for NT
Select checkbox: Run at shutdown only
Under configure for applications: Server control parameters
Program path: C:\Program Files\Tordivel AS\Resuscitator for NT\FindResuscitator.exe
Parameters: -topmost
Select checkbox: Watch this program
Under configure for applications: Scorpion
Program path: C:\Program Files\TORDIVEL AS\Scorpion 6\Scorpion.exe
Parameters: system="Test Prosilica Watchdog" #This is the profile we want to open with scorpion
Start in: C:\Program Files\TORDIVEL AS\Scorpion 6\
Select checkbox: Watch this Program
Select checkbox: Alive check
Example 42: Binary Search¶
Binary Search is a lot faster than linear search
Standard linear search
def linear_search(itemList, searchFor, startIndex=0, endIndex=None):
for item in itemList:
if item == searchFor:
return item
return None
Fast binary search
def binary_search(itemList, searchFor, startIndex=0, endIndex=None):
if endIndex is None:
endIndex = len(itemList)
while startIndex < endIndex:
mid = (startIndex + endIndex)//2
midval = itemList[mid]
if midval < searchFor:
startIndex = mid+1
elif midval > searchFor:
endIndex = mid
else:
return mid
return None
Test script
import random
import time
testList = [n for n in range(10000000)]
testValue = random.randint(0,10000000)
startTime = time.time()
print linear_search(testList, testValue)
elapse = time.time() - startTime
print "Linear [ms]", elapse * 1000.0
startTime = time.time()
print binary_search(testList, testValue)
elapse = time.time() - startTime
print "Binary [ms]", elapse * 1000.0
Console output
6757414
Linear [ms] 326.999902725
6757414
Binary [ms] 0.0
Example 43 - Creating an ordered pointcloud¶
The following script will create an ordered 3D pointcloud
from arrlib import xyzwvfMat
from random import random
cloud = xyzwvfMat(10,10) #1x10 matrix
cloud.tag = 'Example43' #image/overlay name
for r in xrange(10):
for c in range(10):
cloud[r*10+c] = r-5,c-5,random()-0.5,0,0
SetImageMatr('3DModel',cloud)
Example 44 - UDP Socket Communication¶
Script collection demonstrating UDP socket communication in Scorpion.
Central Start:
import socket
receivedFromAddress = None # will be set when receiving data
receivePort = 1501 # listen on this port
sendToAddress = (sendToIPAddress, sendToPort) # target for sending data
udp = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
udp.setsockopt(socket.SOL_SOCKET, socket.SO_REUSEADDR, 1)
udp.bind(('', receivePort))
udp.settimeout(0.01)
Central Stop:
udp = None
Scheduler
# to be called at short intervals from a timer
# ensure no time-consuming tasks are done inside this function.
def Receive():
global udp
global receivedFromAddress
if udp == None:
print 'no udp object'
return
bufsize = 16384
try:
data, receivedFromAddress = udp.recvfrom(bufsize)
except socket.timeout:
return # no data waiting in buffer
except Exception, err:
print 'recv exception:', err
return
print 'Received %d bytes from' % len(data), receivedFromAddress
#use the data string
Send Data
def Send(data):
global udp
sendToIPAddress = '127.0.0.1'
sendToPort = 1500
sendToAddress = (sendToIPAddress, sendToPort)
if udp <> None:
try:
udp.sendto(data, sendToAddress)
except Exception, err:
print 'Send exception: ', err
return
else:
print 'Send: No socket object'
Example 45 - Creating an empty pointcloud¶
The following script will create an empty pointcloud
from arrlib import xyzfVec
cloud=xyzfVec(1) #min vector lengt = 1
cloud.tag='Example45' #image/overlay name
cloud.setLength(0) #set length to 0 - no data
SetImageMatr('3DModel',cloud)